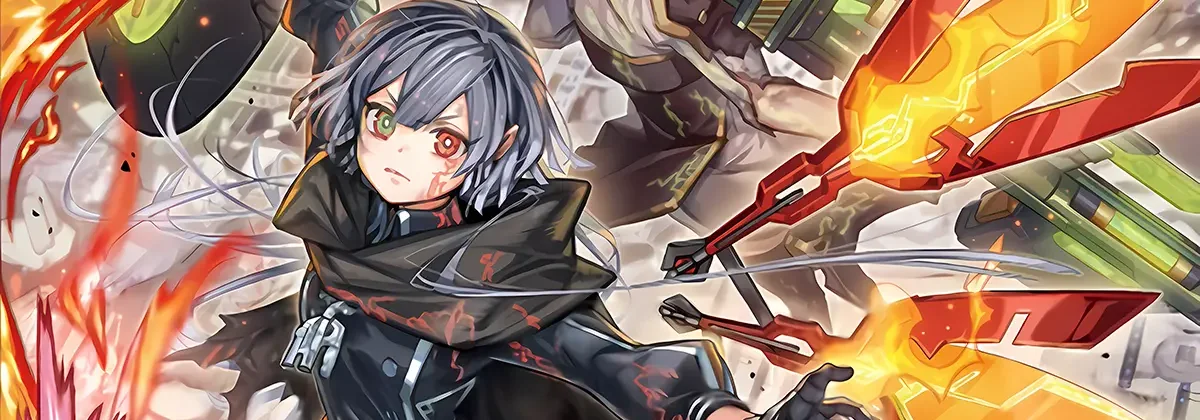
Java 常用工具类
2025年3月4日大约 2 分钟
Java 常用工具类
字符串
String
不可变字符序列
注意事项
- 字符串不可变性导致频繁操作时性能低下
- 推荐场景:常量字符串、键值处理
常用方法
String str = "Hello";
// 获取长度
int len = str.length();
// 截取子串
String sub = str.substring(1,3); // "el"
// 分割字符串
String[] arr = "a,b,c".split(",");
// 替换字符
String newStr = str.replace('l', 'w'); // "Hewwo"
// 拼接字符串
String result = str.concat(" World"); // "Hello World"
StringBuffer
线程安全的可变字符序列
注意事项
- 线程安全但性能较低
- 推荐场景:多线程环境下的字符串操作
常用方法
StringBuffer sb = new StringBuffer();
// 追加内容
sb.append("Hello");
sb.append(123);
// 插入内容
sb.insert(5, " World"); // "Hello World123"
// 反转字符串
sb.reverse();
// 线程安全方法
sb.toString();
StringBuilder
非线程安全的可变字符序列
常用方法
StringBuilder sb = new StringBuilder();
sb.append("Java");
sb.delete(1,3); // "Ja"
sb.replace(0,2, "Py"); // "Pytho"
对比
特性 | String | StringBuffer | StringBuilder |
---|---|---|---|
可变性 | ❌ | ✔️ | ✔️ |
线程安全 | ❌ | ✔️ | ❌ |
性能 | 低 | 中 | 高 |
1
日期时间
Date
(已过时,建议使用 java.time
包)
基本使用
Date now = new Date(); // 当前时间
long time = now.getTime(); // 时间戳
SimpleDateFormat
日期格式化类
使用示例
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
// 日期转字符串
String str = sdf.format(new Date());
// 字符串转日期
Date date = sdf.parse("2023-01-01 12:00:00");
注意事项
- 非线程安全,多线程环境需配合 ThreadLocal 使用
- 格式符号:
yyyy
-年,MM
-月,dd
-日,HH
-小时(24h 制)
Calendar
日期操作类
常用方法
Calendar cal = Calendar.getInstance();
// 获取字段
int year = cal.get(Calendar.YEAR);
// 设置时间
cal.set(2023, Calendar.JANUARY, 1);
// 日期计算
cal.add(Calendar.DAY_OF_MONTH, 5); // 加5天
注意事项
- 月份从 0 开始(0=January)
- 推荐使用
Calendar.getInstance()
获取实例
数学
Number
数值包装类的基类
主要子类
Integer num1 = Integer.valueOf("100");
Double num2 = Double.parseDouble("3.14");
Math
数学计算工具类
常用方法
// 绝对值
Math.abs(-10); // 10
// 最大值
Math.max(5, 10); // 10
// 幂运算
Math.pow(2, 3); // 8.0
// 平方根
Math.sqrt(9); // 3.0
// 随机数 [0,1)
double rand = Math.random();
// 四舍五入
Math.round(3.6); // 4
进阶方法
// 三角函数
Math.sin(Math.PI/2);
// 对数运算
Math.log(Math.E); // 1.0
// 角度转换
Math.toRadians(180); // π 弧度